Introduction to Delete and Truncate
Data management involves two terms that are frequently thrown around: delete and truncate. While they may appear similar at first glance, there are distinct differences that need to be understood to use either effectively. In this article, we’ll look into these differences between delete and truncate as well as their consequences and best practices when used accordingly.
DELETE and TRUNCATE commands are two of the most frequently asked interview questions. Both commands can be used to delete data from databases, though their functionality differs in that delete statements delete without resetting their identities, while truncate commands reset them instead.
What the DELETE command is
DML or data manipulation commands are used to delete records from tables that no longer need to exist in a database. Deleting rows clears them completely out, begets an accurate count of deleted rows, and grants permission for its effective usage. To use this command effectively we require permission from those involved with owning the targeted table to delete it.
The delete command allows us to filter and delete records that meet given criteria from a table using its where clause. However, before running this query we must take precautions as we cannot recover these deleted records with this query.
Database backups allow us to easily restore data whenever it becomes necessary in the future. When acting such as deleting rows from tables, an output showing the number of deleted rows will be produced; alternatively, use the WHERE clause with the DELETE command for targeted deletion of records in tables; however, once this command has been executed it cannot be undone!
Therefore, you should have a database backup before executing this command. Below is the syntax for the DELETE command to remove data from the table: DELETE FROM table_name WHERE condition; Now, understand this with the help of an example. You will create a table named players and insert a few records on the table.
create table players (player_id int, name varchar(20), country varchar(20), goals int); insert into players (player_id, name, country, goals) values (101, ‘Sam’, ‘USA’, 6), (103, ‘Daniel’, ‘England’, 7), (104, ‘Anthony’, ‘France’, 10), (102, ‘Bruno’, ‘Sweden’, 6), (105, ‘Alex’, ‘Wales’, 5), (106, ‘Matt’, ‘Scotland’, 3); Select * from players; Now you must write a query to delete the last record with player_id = 106. Delete from players where player_id = 106;
The SQL query mentioned above will remove the last row, i.e. with player_id = 106, from the players’ table.
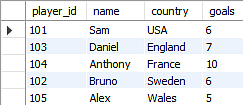
How does the delete command work?
When you execute the delete command, the database engine scans each table for rows that meet a specified condition and removes them from it; if no such condition exists then all rows from that table will be deleted as part of a single SQL statement. To use the delete command in SQL statements:
DELETE FROM table_name WHERE condition;
What the TRUNCATE command is
A truncated statement is a DDL or data definition language command used to delete complete rows without altering table structure. Filtering records through this command isn’t possible due to a lack of log maintenance while performing this operation.
The truncate command allocates pages instead of rows and logs an entry for this action in transaction logs. Furthermore, this command locks pages instead of rows; therefore requiring fewer locks and resources than its counterpart.
Note that, when using the truncate statement on tables referencing foreign keys or participating in an indexed view, we cannot use its WHERE clause; hence you cannot filter records with it and it doesn’t register an audit trail for deletions during execution.
Executing this command locks pages rather than rows; as a result, it uses fewer locks and resources. The syntax for using TRUNCATE to delete data from a table: TRUNCATE TABLE table_name
Here, you’ll use the truncate command to delete all records from the table players. With this SQL query, all records will be removed. Now run this query to verify the table structure: “Describe Players”.
As you can see above, though the players’ table has been truncated, the table structure remains intact.
How does the truncate command work?
When you execute the truncate command, the database engine deallocates all the data pages associated with the table, releasing the storage space. It resets the identity value to its original seed value.
The truncate command can be executed using a simple SQL statement:
TRUNCATE TABLE table_name;
Key differences between DELETE and TRUNCATE
The following points explain the differences between the delete and truncate commands:
- The DELETE command is used to delete all or some records in a table. TRUNCATE will remove entire rows.
- DELETE is only a DML Command, as it modifies table data. TRUNCATE on the other hand is a DDL Command.
- The DELETE command allows you to filter records/tuples using the WHERE clause. The TRUNCATE command, however, does not permit the WHERE condition, so we can’t filter rows when truncating.
- DELETE triggers all delete triggers in the table. The truncate function does not fire any triggers because it doesn’t operate on individual columns.
- DELETE deletes rows one by one from the table in the order they were processed. TRUNCATE, on the other hand, deletes entire tables at once instead of rows.
- The DELETE statement deletes only records and does not reset ‘s table identity. TRUNCATE resets a table’s identity.
- DELETE requires more database resources and locks because it locks each row deleted. TRUNCATE, on the other hand, locks the page of data before deleting it. This requires fewer locks and resources.
- TRUNCATE records each page’s transaction log. DELETE makes a record in the log of each row deleted.
- The TRUNCATE command is faster than the DELETE command as it deallocates data pages instead of rows and records data pages instead of rows in the transaction log.
- We cannot recover deleted records once they have been deleted using TRUNCATE. We can, however, recover deleted data that we removed using the DELETE command.
- The main difference between delete (remove) and truncate (truncate) is their impact on the table data. The delete command only removes the specified rows and leaves the other data in your table intact. The truncate option, on the other hand, removes all data from the table.
- The performance between delete and truncate commands is significantly different. Depending on the size and complexity of the table, the delete command may take a while to execute. The truncate option is faster and more efficient than the delete command, especially when dealing with large tables.
- The logging and recovery mechanisms are another significant difference between delete (and truncate). The delete command creates a log entry in the transaction logs for every row that is removed from the table. These entries are useful in the event of a database or system failure. The truncate commands do not create log entries. This makes it impossible to recover the data after it has been truncated.
Permissions
Delete and truncate commands have different permissions requirements. The delete command requires deleting permission on the table, while the truncate command requires altering permission on the table.
Delete and Truncate Comparison Chart
Comparison Basis | DELETE | TRUNCATE |
---|---|---|
Definition |
The delete statement is used to remove single or multiple records from an existing table depending on the specified condition. | The truncate command removes the complete data from an existing table but not the table itself. It preserves the table structure or schema. |
Language |
It is a DML (Data Manipulation Language) command. | It is a DDL (Data Definition Language) command. |
WHERE |
It can use the WHERE clause to filter any specific row or data from the table. | It does not use the WHERE clause to filter records from the table. |
Permission |
We need to have DELETE permission to use this command. | We need to have ALTER permission to use this command. |
Working |
This command eliminates records one by one. | This command deletes the entire data page containing the records. |
Lock |
It will lock the row before deletion. | It will lock the data page before deletion. |
Table Identity |
This command does not reset the table identity because it only deletes the data. | It always resets the table identity. |
Transaction |
It maintains transaction logs for each deleted record. | It does not maintain transaction logs for each deleted data page. |
Speed |
Its speed is slow because it maintains the log. | Its execution is fast because it deletes entire data at a time without maintaining transaction logs. |
Trigger |
This command can also activate the trigger applied to the table and cause it to fire. | This command does not activate the triggers applied on the table to fire. |
Restore |
It allows us to restore the deleted data by using the COMMIT or ROLLBACK statement. | We cannot restore the deleted data after executing this command. |
Indexed view |
It can be used with indexed views. | It cannot be used with indexed views. |
Space |
The DELETE statement occupies more transaction space than truncate because it maintains a log for each deleted row. | The TRUNCATE statement occupies less transaction space because it maintains a transaction log for the entire data page instead of each row. |
When to use Delete and Truncate?
Both delete and truncate commands have their use cases, depending on the scenario and the objective. The delete command is best suited for removing specific rows from a table based on a condition. For example, if you want to remove all the rows with a particular customer name from the customer table, you would use the delete command.
On the other hand, the truncate command is best suited for clearing all the data from a table quickly. If you want to delete all the records from the customer table, you can use the truncate command instead of deleting each row individually using the delete command.
Best practices when using Delete and Truncate
When using the Delete and Truncate commands, it’s essential to follow certain best practices to avoid unintended consequences.
Some of these best practices are:
- Always take a backup of the table before executing the delete or truncate command.
- Test the command in a development or staging environment before executing it in the production environment.
- Always specify a condition when using the delete command to avoid deleting all the rows unintentionally.
- Avoid using the truncate command on tables with foreign keys or constraints as it can cause data integrity issues.
Consequences of Misusing Delete and Truncate
Misusing either of the delete and truncate commands can have severe repercussions, including data loss or corruption. If you use delete without specifying a condition, it will delete all rows from a table, creating irreparable damage. Likewise, using the truncate command on tables with foreign keys or constraints may create data integrity problems that lead to data integrity issues.
Final verdict about Delete and Truncate
Understanding the difference between Delete and Truncate commands helps SQL developers safely remove rows from a table. Be careful when using TRUNCATE; it erases all records in its wake! This tutorial can also assist novice users in understanding what these two commands do. If you have any inquiries related to our content on SQL DELETE and SQL TRUNCATE, feel free to post in our comments section below; our experts will gladly review them and assist in solving them ASAP!
Delete and truncate are two SQL commands used to remove data from database tables. Delete removes rows based on certain conditions, while truncate removes everything in its entirety from a table. It is essential to understand these two commands’ differences and the potential unintended repercussions for the successful use of each.